powershell
-
I had to run the installer twice before it worked
-
gpodder picked up all my subscriptions from gpodder.net, which was handy, because I’d forgotten to export the opml
-
I had to log out and back in again to get gpodder to save to the folder I specified
-
Spotify seems identical. I use custom order, which I wasn’t expecting to be there in the Linux version….I’m wondering whether it’s actually the windows version running under wine
-
it’s a shame PSClock won’t run
-
I installed the Mate desktop…I very much prefer that to the default Ubuntu one
-
I haven’t got gvim set up yet
-
or VS Code
-
or any powershell stuff beyond installing it
I almost certainly spent longer looking for this today than it would have taken to re-do it. My most used powershell debugger commands. I will redo it at some stage and add in:
get-psbreakpoint | disable-psbreakpoint
…and this cmdlet what I wrote: Get-MuNonStandardVariables.ps1
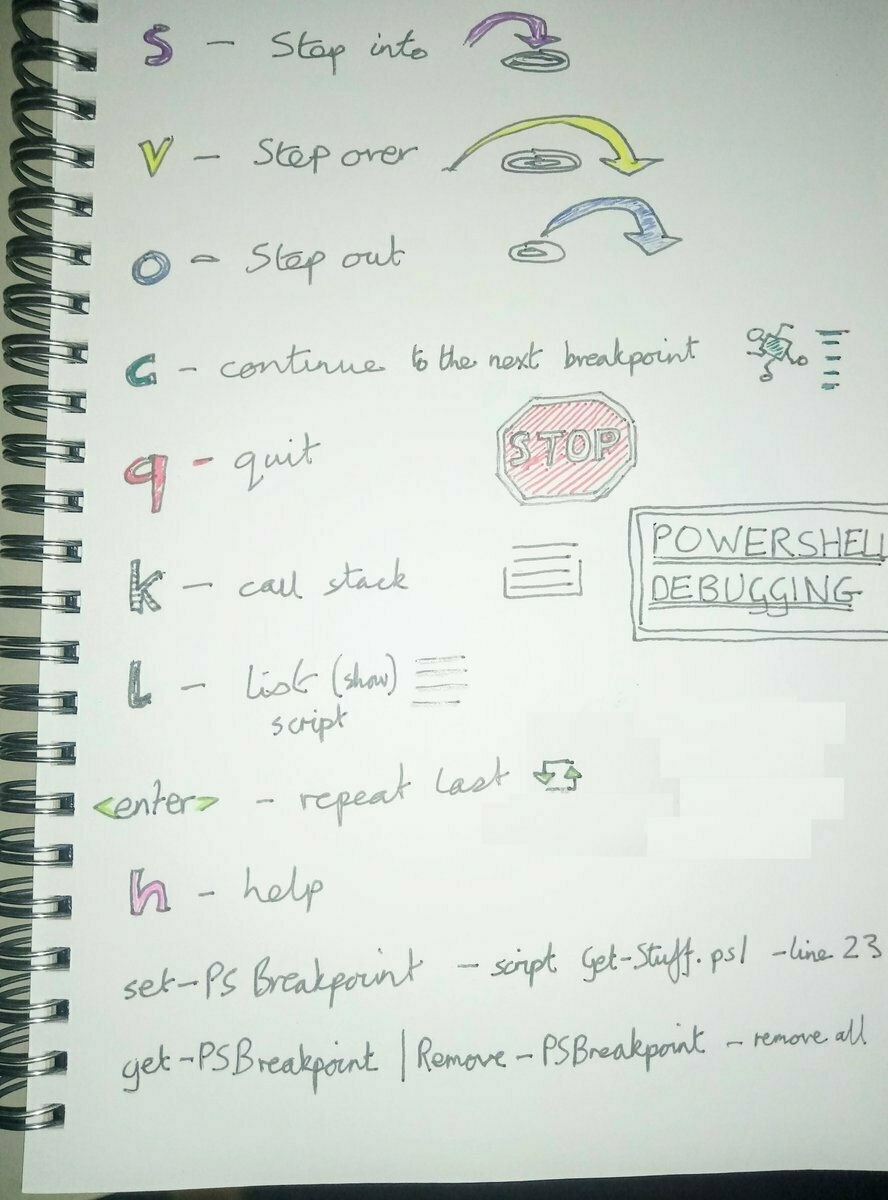
Using Out-Gridview to interactively select one item from a collection
I’m not sure I’ve ever had cause to do this before…but it’s quite handy.
I’m writing an add-on to Spotishell, a powershell module for mucking about with Spotify. I’m searching for a song and then I want to manually select the one that I think is the best version to include on a playlist.
Out-Gridview turns out to be perfect for this.
The code is:
$SelectedTrack = $CollectionOfTracks |
select-object track,
artist,
album,
released,
trackid |
Out-GridView -OutputMode Single
This returns the one track into the $SelectedTrack variable.
<img src=“https://cdn.uploads.micro.blog/139254/2025/screenshot-2025-03-31-175209.png" width=“600” height=“342” alt=“A computer window displays a list with details about tracks titled “The Sick Bed of Cuchulainn” by The Pogues, including albums, release years, and unique identifiers.">
Unshortening a shortened URL with powershell....as of February 2025 anyway
There are a bunch of pages on the internet giving code to expand shortened URLs, but none of the ones I tried seem to work.
I suspect this might be because it’s changed over time….but this seems to work…atm!
((Invoke-WebRequest -UseBasicParsing βUri 'https://bit.ly/3D0xStd').baseresponse).RequestMessage.RequestUri.AbsoluteUri
Correctly gives:
https://newsthump.com/2025/02/16/master-negotiator-donald-trump-to-end-ukraine-war-by-simply-giving-russia-everything-it-wants/
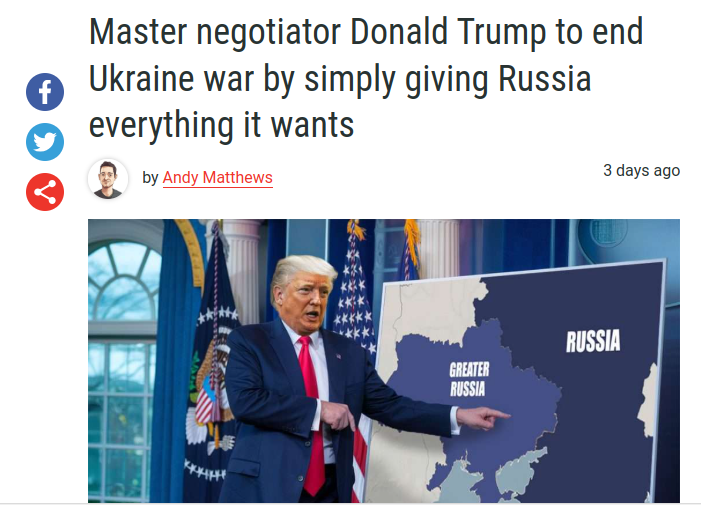
I forgot to remember that setting:
$ErrorView = ‘NormalView’
….gives you useful stuff like the line number of the code that’s failed in Powershell 7
I need to have a play with this sooner rather than later
Microsoft Entra blog - Microsoft Entra PowerShell module now generally available
How to delete from a tweets micro.blog with powershell
These were my last three tweets on the blog…and, I think, the last tweets I posted
invoke-restmethod https://micro.blog/micropub?mp-destination=https://mattypenny-tweets.micro.blog`&q=source`&offset=0`&limit=3 `
-Method get -Header $RestMethodHeaders |
select -expand items |
select -expand properties |
select url,content
url content
--- -------
{https://mattypenny-tweets.micro.blog/2022/12/31/1609327355398443008.html} {Happy new year to you and yours!}
{https://mattypenny-tweets.micro.blog/2022/12/24/1606763511543209984.html} {God bless us, every one}
{https://mattypenny-tweets.micro.blog/2022/12/23/1606293329544548352.html} {My word of the day today is 'myrrh'.}
So, to delete a specific tweet from the micro.blog….
Set up the headers
$RestMethodHeaders = @{
"Authorization" = "Bearer $BlogToken"
}
Delete the ‘happy new year’ one
invoke-restmethod https://micro.blog/micropub?mp-destination=https://mattypenny-tweets.micro.blog`&url=https://mattypenny-tweets.micro.blog/2022/12/31/1609327355398443008.html`&action=delete `
-Method post `
-Header $RestMethodHeaders
…and abracadbra
invoke-restmethod https://micro.blog/micropub?mp-destination=https://mattypenny-tweets.micro.blog`&q=source`&offset=0`&limit=3 -Method get -Header $RestMethodHeaders | select -expand items | select -expand properties | select url,content
url content
--- -------
{https://mattypenny-tweets.micro.blog/2022/12/24/1606763511543209984.html} {God bless us, every one}
{https://mattypenny-tweets.micro.blog/2022/12/23/1606293329544548352.html} {My word of the day today is 'myrrh'.}
{https://mattypenny-tweets.micro.blog/2022/12/22/1605866305227087873.html} {My word of the day today is 'eggnog'.}
βPowershell to get a list of micro.blog posts
Set your headers:
$headers = @{
"Authorization" = "Bearer $Token"
"Content-Type" = "application/json"
}
…where $Token is retrieved from micro.blog
Then:
$Uri = https://micro.blog/micropub?q=source`&mp-destination=https%3a%2f%2fmattypenny-tweets.micro.blog%2f
$i = Invoke-RestMethod -Uri $Uri-Method Get -Headers $headers
….replacing mattypenny-tweets.micro.blog with the name of your blog
Then:
$i | select -expand items | select -expand properties | select post-status,published ,content
…which gives the below.
I think you can put start and end dates on the query….I’ll add that in later
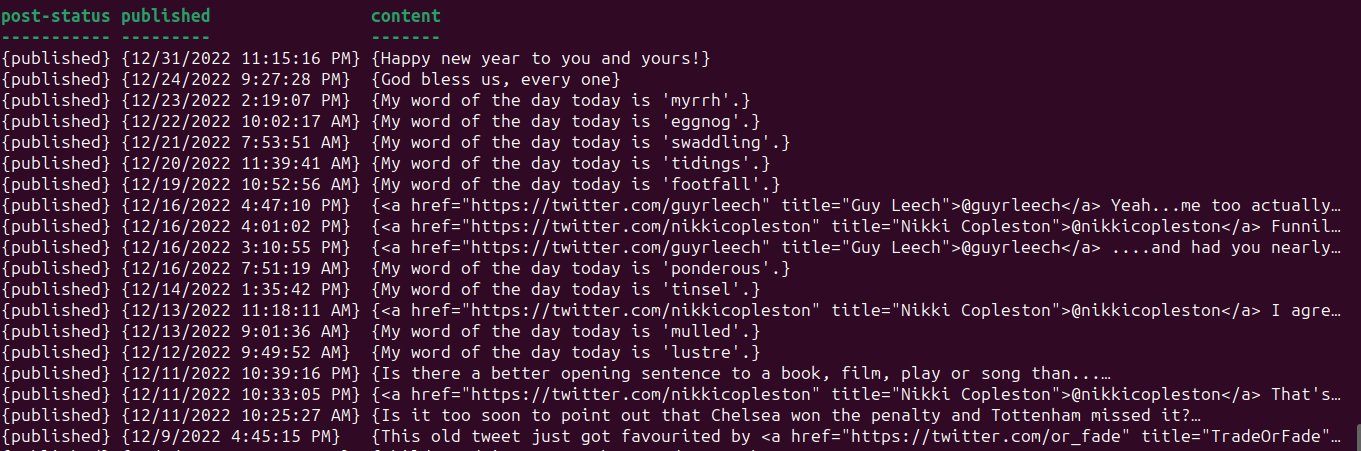
I’m mucking about with the idea of doing some #sketchnote pages on Powershell basics
#Powershell
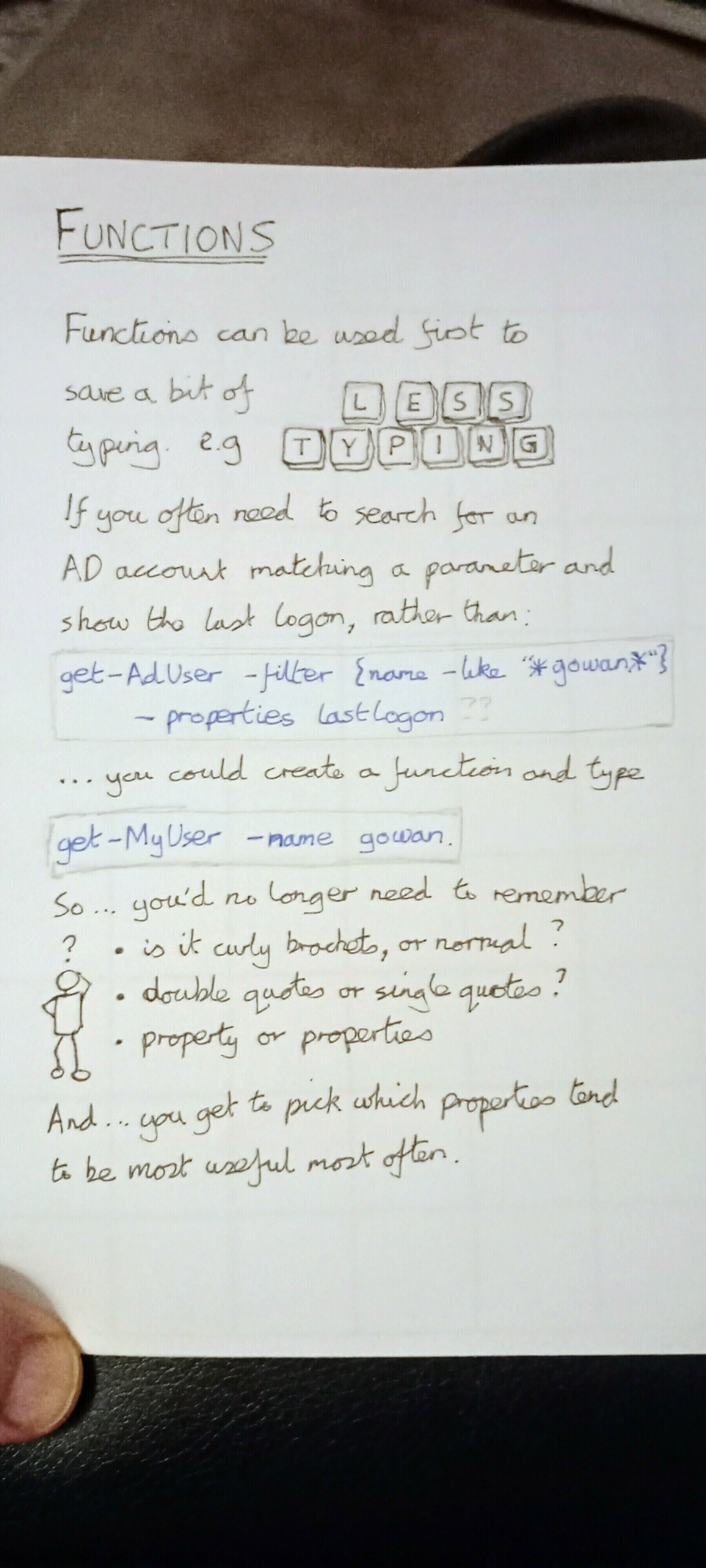
How to post to a test instance of micro.blog with powershell
Source the micro.blog token
$BlogToken = $(import-csv /stuff/GeneralParameters.csv | ? parameter -eq 'BlogToken').value
$headers = @{
"Authorization" = "Bearer $BlogToken"
}
Get details for the micro.blog
$response = Invoke-RestMethod -Uri "https://micro.blog/micropub?q=config" -Headers $headers
This is the bit to get the uid of the test blog - mine is a fairly simple variation on the name of the main blog…as below. The URLEncode returns ‘https%3a%2f%2fmattypenny-test.micro.blog%2f’
$response | select -ExpandProperty destination
$MpDestination = [System.Web.HttpUtility]::UrlEncode("https://mattypenny-test.micro.blog/")
build the Uri to upload to
$mediaEndpoint = $response."media-endpoint"
$Uri ="${mediaEndpoint}?mp-destination=$MpDestination"
Identify the file to upload
$form = @{
file = Get-Item '/home/matty/working/spotify/images/Bethany Eve - Emerald City.png'
}
Upload it
Invoke-RestMethod -Uri $Uri -Method Post -Headers $headers -Form $form
And….hey presto
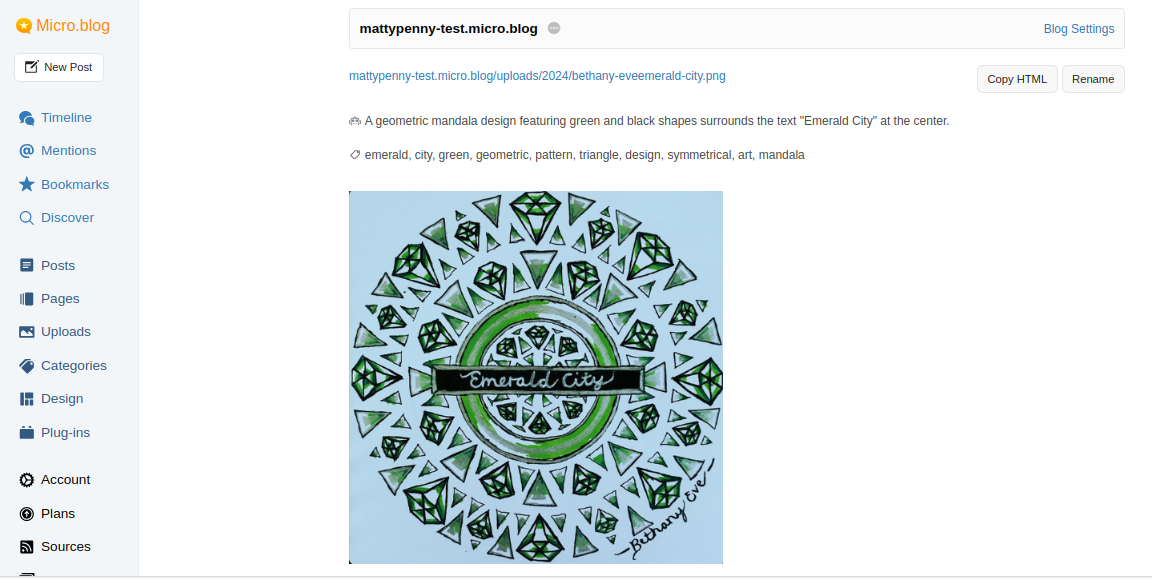
I’m enjoying the #Powershell tips module. I’ve already learnt a couple of things….and I’ve been using Powershell for about 103 years
GitHub - deadlydog/PowerShell.tiPS: PowerShell tips delivered straight to your terminal π»
There’s a video about contributing here:
PowerShell tiPS module - How to add your PowerShell tips to share them with the world
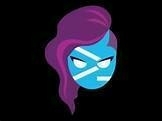
Powershelling my twitter archive
I’ve been looking at my twitter archive, with a view to importing bits of it into micro.blog (the automatic import didn’t quite work…I’m blaming Musk!)
I’m using PowerShell
Anyway so far I’ve learnt two things
You have to take this bit out of the first line of the tweets.js file for convertfrom-json to work:
window.YTD.tweets.part0 =
Some tweets seem to be truncated with a special character that looks like three dots….but this seems to mainly but not exclusively be retweets and the display url
"created_at" : "Sun Apr 16 12:55:38 +0000 2017",
"favorited" : false,
"full_text" : "RT @SkrMkrSean: I'm at Ashton United v Blyth Spartans and all the Blyth are dressed as Mexicans holding up a wall banner behind a guy in aβ¦",
I linux-ified my aging windows laptop over the weekend
All good, in general, and I’ll be able to get another couple of years out of it
I found that:
……but it’s all good
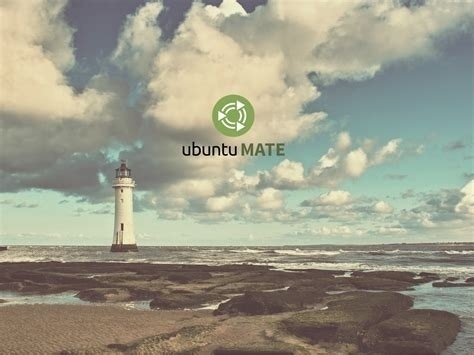
“At this point, I’m pretty sure, that if I lost my .zsh_history I probably wouldn’t be able to do my job anymore. Most of the commands I run are really just CTRL+R plus minor edits.” [Shell History Is Your Best Productivity Tool - Martin Heinz] (https://martinheinz.dev/blog/110?utm_source=tldrnewsletter)
Me too, although replacing .zsh_history with whatever’s in $(Get-PSReadLineOption).HistorySavePath
How to continuously run a set of Pester tests from the commandline
while ($(get-date).hour -lt 19) {invoke-pester .\tests-folder\*.tests.ps1 -output Normal 5>c:\temp\debug_output.txt }
I imagine you can do this from within VS Code, but I sometimes have this running on a 2nd screen while I’m coding.
How to set default parameters for other people's Powershell cmdlets
Not exactly #TodayILearned because I vaguely remember learning that you can do this, but had never got around to doing so.
This is how you can set a default parameter in Powershell. As far as I know, it works with any cmdlet. I’m using it here to set the default for Pester to show me all the results of all the tests….because I love seeing that sea of green :)
$PSDefaultParameterValues += @{ 'Invoke-Pester:Output' = 'Detailed' }
#Powershell
I need to decide whether I’m going to use $SplatParams or $SplatParameters….and then get that tattoo-ed on the inside of my eyelids or something
#Powershell
#TodayILearned that one way of getting a list of data available from Microsoft Graph API is to type ‘graph.microsoft.com/v1.0/user… into Graph Explorer, and see what show’s up in the Dropdown
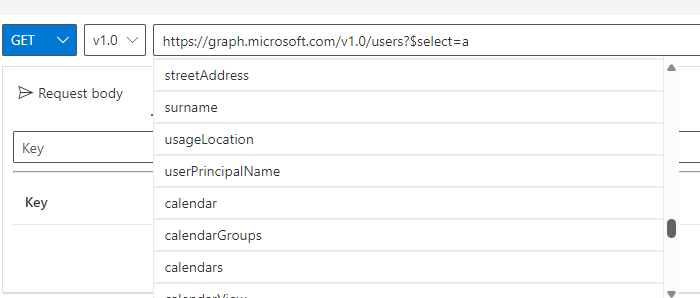
Vim commands to write out parameter list
Executive summary :)
For each line
0
D
PP
From the colon prompt:
%s/^ */ write-dbg "`
%s/ *\$/: <\$
%s/$/>"
%s/,//g
The gory details
Starting with a parameter clause like this:
Param(
$Parameter1,
$Parameter2,
$Parameter3,
$Parameter4
)
The manual bits
First, take off the ‘Param(i’, and the bracket at the end
Second, for each line do this:
0
D
PP
… to get:
$Parameter1, $Parameter1,
$Parameter2, $Parameter2,
$Parameter3, $Parameter3,
$Parameter4 $Parameter4
The colon prompt bits
Replace spaces at the front with the write-dbg, a double-quote, and then a backtick. The backtick is because I need to escape the name of the variable
:%s/^ */ write-dbg "`
This gives:
write-dbg "`$Parameter1, $Parameter1,
write-dbg "`$Parameter2, $Parameter2,
write-dbg "`$Parameter3, $Parameter3,
write-dbg "`$Parameter4 $Parameter4
Replace the spaces between the repeated variables with ‘: <’
:%s/ *\$/: <\$
….giving:
write-dbg "`$Parameter1,: <$Parameter1,
write-dbg "`$Parameter2,: <$Parameter2,
write-dbg "`$Parameter3,: <$Parameter3,
write-dbg "`$Parameter4: <$Parameter4
End the string:
%s/$/>"
….which gives:
write-dbg "`$Parameter1,: <$Parameter1,
write-dbg "`$Parameter2,: <$Parameter2,
write-dbg "`$Parameter3,: <$Parameter3,
write-dbg "`$Parameter4: <$Parameter4
Finally get rid of the commas
%s/,//g
Leaving:
write-dbg "`$Parameter1: <$Parameter1>"
write-dbg "`$Parameter2: <$Parameter2>"
write-dbg "`$Parameter3: <$Parameter3>"
write-dbg "`$Parameter4: <$Parameter4>"
I’ve been told that, ergonomically, the top of your monitor should be level with your eyes.
I’m sure this is good advice, but I’m wondering if it applies to people who live on the command line, because on the command line stuff tends to happen at the bottom of the screen #Powershell
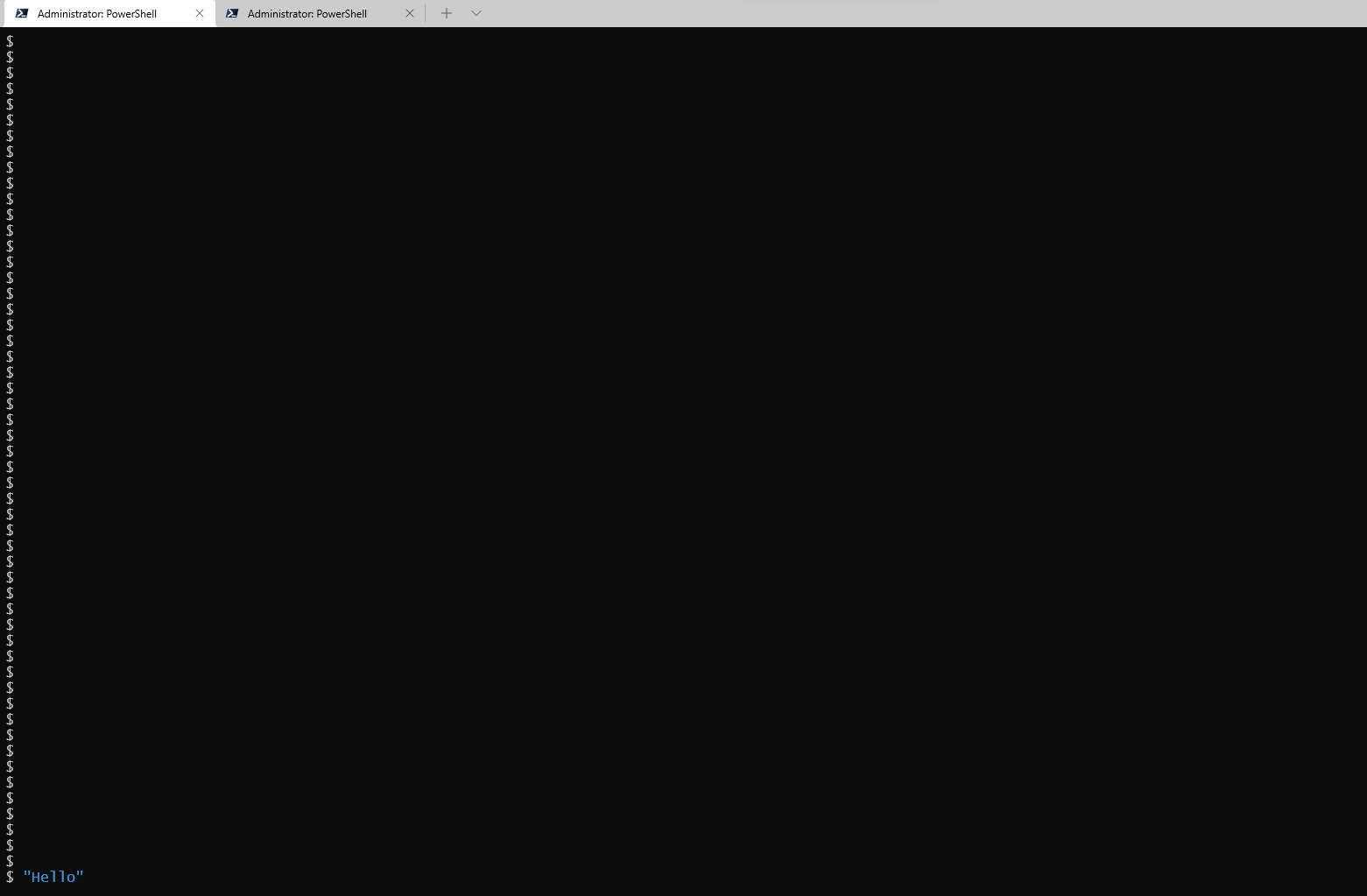
How to post to a test micro.blog from powershell
Sorry about the formatting of this post…I’m having trouble getting markdown not to convert the url to markdown links, even within code blocks
There’s a bit more detail on posting generally from powershell at mattypenny.micro.blog/2024/02/1…
This is the standard bit
$Token = 'whatever-the-token-is' | ConvertTo-SecureString -AsPlainText -Force
$Body = @{ content = 'Testing again. 1 , 2, 1, 2' h = 'entry' 'post-status' = 'draft' }
You then put ‘?mp-destination=’ followed by the URL of the test micro.blog on the end of the base URL as in the gist below. (The markdown conversion is nausing up the URL if I type it in here - sorry!
invoke-restmethod micro.blog/micropub -Method post -Authentication Bearer -Token $Token -Body $Body
url preview edit
mattypenny-test.micro.blog/2024/02/1… micro.blog/account/p… micro.blog/account/p…
The output confirms that the post has gone to mattypenny-test.micro.blog
